Introduction
Motion sensing is a fundamental aspect of many robotics and electronics projects, enabling devices to perceive and respond to their surroundings. The MPU-6050 Accelerometer and Gyroscope module with Arduino is a versatile sensor that combines both accelerometer and gyroscope capabilities, making it an essential component for projects requiring accurate motion detection and orientation tracking.
In this comprehensive guide, we will explore the working principle and features of the MPU-6050 sensor module. Additionally, we will learn how to interface it with an Arduino UNO to create a motion-sensing system. By the end of this tutorial, you will be equipped with the knowledge and skills to integrate the MPU-6050 sensor module into your projects, enhancing their motion-sensing capabilities. So, let’s embark on this journey of motion sensing with Arduino and uncover the potential of the MPU-6050 Accelerometer and Gyroscope Module!
Hardware Required
You will require the following Hardware Components for interfacing MPU-6050 Accelerometer and Gyroscope module with Arduino.
Components | # | Buy From Amazon |
---|---|---|
Arduino UNO | 1 | Buy Now |
MPU-6050 Accelerometer and Gyroscope Sensor | 1 | Buy Now |
9v DC Adapter (Optional) | 1 | Buy Now |
Jumper Wires | Few | Buy Now |
Breadboard | 1 | Buy Now |
What is MPU-6050 Sensor?
The MPU-6050 Accelerometer and Gyroscope Module is a compact sensor that combines a three-axis accelerometer and a three-axis gyroscope on a single chip. It measures linear acceleration and angular velocity along three axes, enabling accurate motion detection and orientation tracking. The accelerometer measures changes in velocity, allowing the sensor to detect motion, while the gyroscope measures angular velocity, helping determine the orientation of the module. This combined functionality makes the MPU-6050 an excellent choice for projects involving robotics, drone stabilization, gesture recognition, and more.
Pinout

MPU6050 Pinout Configuration
Pin Name | Description |
---|---|
Vcc | Provides power for the module, can be +3V to +5V. Typically +5V is used |
Ground | Connected to Ground of system |
(SCL) Serial Clock | Used for providing clock pulse for I2C Communication |
(SDA) Serial Data | Used for transferring Data through I2C communication |
(XDA) Auxiliary Serial Data | Can be used to interface other I2C modules with MPU6050. It is optional |
(XCL) Auxiliary Serial Clock | Can be used to interface other I2C modules with MPU6050. It is optional |
AD0 | If more than one MPU6050 is used a single MCU, then this pin can be used to vary the address |
(INT) Interrupt | Interrupt pin to indicate that data is available for MCU to read. |
Specifications
- Axes of Measurement: The MPU-6050 module can measure motion along three axes (X, Y, Z) for both accelerometer and gyroscope data.
- Communication Interface: The module communicates with microcontrollers like Arduino through I2C (Inter-Integrated Circuit) communication protocol.
- Operating Voltage: The module operates within the voltage range compatible with Arduino UNO.
- Compact Size: The small form factor of the MPU-6050 module allows for easy integration into projects.
Features
- Combined Motion Sensing: The module combines accelerometer and gyroscope data, providing comprehensive motion-sensing capabilities.
- Fast Response Time: The MPU-6050 module exhibits a fast response time, enabling real-time motion detection and orientation tracking.
- Digital Temperature Sensor: The module includes an onboard digital temperature sensor for accurate temperature measurements.
Circuit Diagram
The following circuit shows you the connection of the Interfacing MPU-6050 Accelerometer and Gyroscope Sensor with Arduino Please make the connection carefully
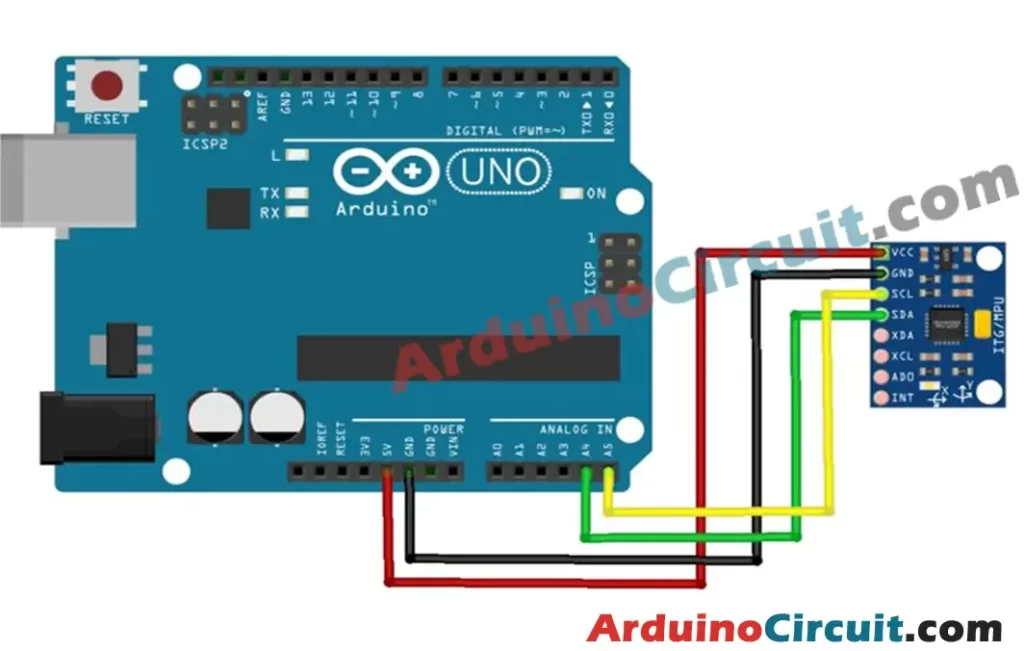
Circuit Connections
Arduino | MPU6050 Sensor |
---|---|
+5V | VCC Pin |
GND | GND Pin |
A4 | SDA |
A5 | SCL |
Installing Arduino IDE Software
First, you will require to Download the updated version of Arduino IDE Software and Install it on your PC or laptop. if you Learn How to install the Arduino step-by-step guide then click on how to install Arduino Button given Blow
Installing Libraries
Now when you are Ready to upload the code, to the Arduino Board you will need first to add the Following Libraries in Arduino, If you Learn How to add the library in the Arduino step-by-step guide click on how to install the library Button given Blow
Code
//For more Projects: www.arduinocircuit.com
#include <Wire.h>
#include <MPU6050.h>
MPU6050 mpu;
void setup()
{
Serial.begin(115200);
Serial.println("Initialize MPU6050");
while(!mpu.begin(MPU6050_SCALE_2000DPS, MPU6050_RANGE_2G))
{
Serial.println("Could not find a valid MPU6050 sensor, check wiring!");
delay(500);
}
// If you want, you can set accelerometer offsets
// mpu.setAccelOffsetX();
// mpu.setAccelOffsetY();
// mpu.setAccelOffsetZ();
checkSettings();
}
void checkSettings()
{
Serial.println();
Serial.print(" * Sleep Mode: ");
Serial.println(mpu.getSleepEnabled() ? "Enabled" : "Disabled");
Serial.print(" * Clock Source: ");
switch(mpu.getClockSource())
{
case MPU6050_CLOCK_KEEP_RESET: Serial.println("Stops the clock and keeps the timing generator in reset"); break;
case MPU6050_CLOCK_EXTERNAL_19MHZ: Serial.println("PLL with external 19.2MHz reference"); break;
case MPU6050_CLOCK_EXTERNAL_32KHZ: Serial.println("PLL with external 32.768kHz reference"); break;
case MPU6050_CLOCK_PLL_ZGYRO: Serial.println("PLL with Z axis gyroscope reference"); break;
case MPU6050_CLOCK_PLL_YGYRO: Serial.println("PLL with Y axis gyroscope reference"); break;
case MPU6050_CLOCK_PLL_XGYRO: Serial.println("PLL with X axis gyroscope reference"); break;
case MPU6050_CLOCK_INTERNAL_8MHZ: Serial.println("Internal 8MHz oscillator"); break;
}
Serial.print(" * Accelerometer: ");
switch(mpu.getRange())
{
case MPU6050_RANGE_16G: Serial.println("+/- 16 g"); break;
case MPU6050_RANGE_8G: Serial.println("+/- 8 g"); break;
case MPU6050_RANGE_4G: Serial.println("+/- 4 g"); break;
case MPU6050_RANGE_2G: Serial.println("+/- 2 g"); break;
}
Serial.print(" * Accelerometer offsets: ");
Serial.print(mpu.getAccelOffsetX());
Serial.print(" / ");
Serial.print(mpu.getAccelOffsetY());
Serial.print(" / ");
Serial.println(mpu.getAccelOffsetZ());
Serial.println();
}
void loop()
{
Vector rawAccel = mpu.readRawAccel();
Vector normAccel = mpu.readNormalizeAccel();
Serial.print(" Xraw = ");
Serial.print(rawAccel.XAxis);
Serial.print(" Yraw = ");
Serial.print(rawAccel.YAxis);
Serial.print(" Zraw = ");
Serial.println(rawAccel.ZAxis);
Serial.print(" Xnorm = ");
Serial.print(normAccel.XAxis);
Serial.print(" Ynorm = ");
Serial.print(normAccel.YAxis);
Serial.print(" Znorm = ");
Serial.println(normAccel.ZAxis);
delay(10);
}
Applications
- Robotics: The MPU-6050 module widely uses in robotics for precise motion control and stabilization.
- Drone Stabilization: In drones and quadcopters, the module helps maintain stable flight by detecting changes in orientation.
- Gesture Recognition: This module uses in projects involving gesture recognition and control.
- Virtual Reality (VR) Applications: MPU-6050 enables tracking of head movement and orientation in VR applications.
- Motion-Activated Devices: this module uses in motion-activated switches, toys, and interactive installations.
Conclusion
You have now familiarized yourself with the MPU-6050 Accelerometer and Gyroscope Module and its potential in motion-sensing projects. By interfacing the module with Arduino UNO, you can create robotics, drones, and interactive projects that respond to changes in motion and orientation. The module’s combined accelerometer and gyroscope capabilities make it a valuable tool for motion detection and control. Armed with this knowledge, you can explore the world of motion sensing and implement the MPU-6050 Accelerometer and Gyroscope Module in your projects to bring them to life with precise motion capabilities. So, ignite your creativity and let the MPU-6050 module guide you toward innovative motion-sensing solutions.